Considering donating if you found my post helpful 😊
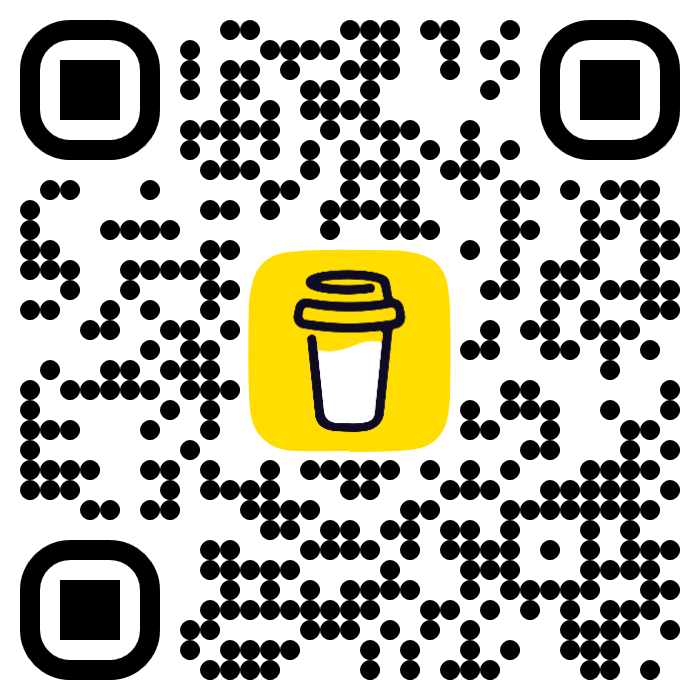
Contents
hide
Description
I was trying to use cURL in PHP, but somehow HTTP code returns 0 instead of any valid response code such as 200, 500, or 403.
This is the code I am using
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $this->api . '/login');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'POST');
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
curl_setopt($ch, CURLOPT_HEADER, true);
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Referer: ' . $this->api
]);
$httpcode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
$r = curl_exec($ch);
curl_close($ch);
if ($httpcode != 200) {
throw new Exception('API returned HTTP code of ' . $httpcode);
}
Some of you might noticed, the error lies in line 11 – $httpcode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
That line should be called after executing the cURL.
Solution
The solution is quite obvious. I need to call curl_getinfo
after curl_exec
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $this->api . '/login');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'POST');
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
curl_setopt($ch, CURLOPT_HEADER, true);
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Referer: ' . $this->api
]);
$r = curl_exec($ch);
$httpcode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
curl_close($ch);
if ($httpcode != 200) {
throw new Exception('API returned HTTP code of ' . $httpcode);
}
Problem solved!
Considering donating if you found my post helpful 😊
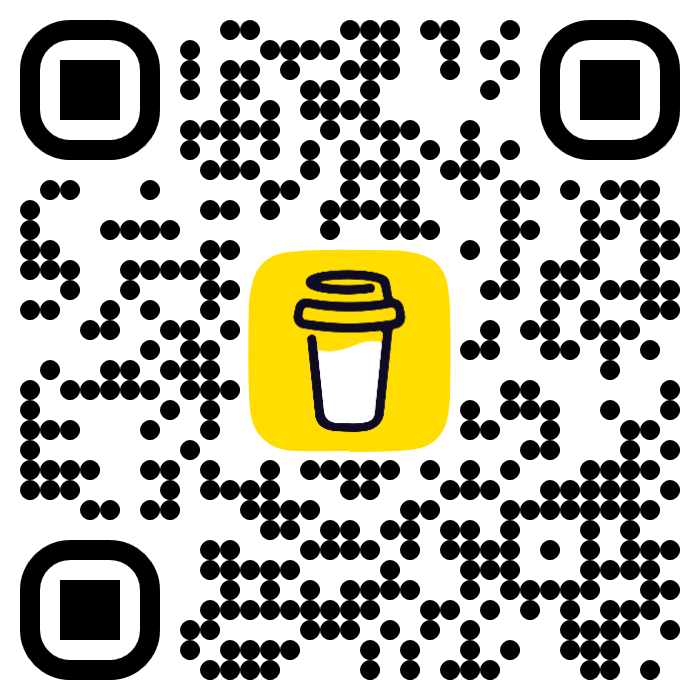
[…] + Read More […]